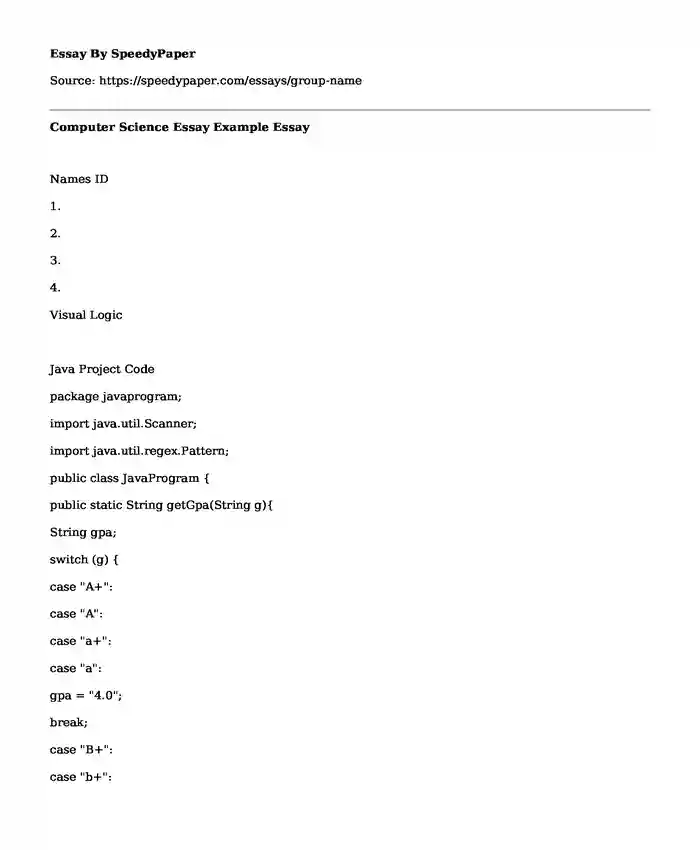
Type of paper:Â | Essay |
Categories:Â | Computer science Software |
Pages: | 3 |
Wordcount: | 560 words |
Names ID
1.
2.
3.
4.
Visual Logic
Java Project Code
package javaprogram;
import java.util.Scanner;
import java.util.regex.Pattern;
public class JavaProgram {
public static String getGpa(String g){
String gpa;
switch (g) {
case "A+":
case "A":
case "a+":
case "a":
gpa = "4.0";
break;
case "B+":
case "b+":
gpa = "3.75";
break;
case "B":
case "b":
gpa = "3.5";
break;
case "C+":
case "c+":
gpa = "3.0";
break;
case "C":
case "c":
gpa = "2.5";
break;
case "D+":
case "d+":
gpa = "2.0";
break;
case "D":
case "d":
gpa = "1.0";
break;
case "F":
case "f":
gpa = "0.0";
break;
default:
gpa = "Sorry,your grade entry is not supported, please check your entry and try again.";
break;
}
return gpa;
}
public static void main(String[] args) {
Scanner reader = new Scanner(System.in); // Reading from System.in
System.out.print("please enter your grade: ");
String grade = reader.next();
String gpa;
gpa = getGpa(grade);
System.out.println("The GPA for grade " +grade.toUpperCase() + " is "+ gpa);
System.out.println();
System.out.print("please enter Course Code1: ");
String code1 = reader.next();
System.out.print("please enter credit hours for Course Code1-"+code1+":");
String credit1 = reader.next();
System.out.print("please enter Grade for course code1-"+code1+":");
String courseGrade1 = reader.next();
System.out.println();
System.out.print("please enter Course Code2: ");
String code2 = reader.next();
System.out.print("please enter credit hours for Course Code2-"+code2+":");
String credit2 = reader.next();
System.out.print("please enter Grade2 for Course Code2-"+code2+":");
String courseGrade2 = reader.next();
System.out.println();
System.out.print("please enter Course Code3: ");
String code3 = reader.next();
System.out.print("please enter credit hours for Course Code3-"+code3+":");
String credit3 = reader.next();
System.out.print("please enter Grade for Course Code3-"+code3+":");
String courseGrade3 = reader.next();
System.out.println(courseGrade3);
//Check to ensure valid input of hours
String pattern = "[+-]?[\\d,]+\\.?\\d+";
if(Pattern.matches(pattern, credit1) == true){
System.out.println();
System.out.println("You entered INVALID credit hour for course code1, please check and retry again.");
return;
}
if(Pattern.matches(pattern, credit2) == true){
System.out.println();
System.out.println("You entered INVALID credit hour for course code2, please check and retry again.");
return;
}
if(Pattern.matches(pattern, credit3) == true){
System.out.println();
System.out.println("You entered INVALID credit hour for course code3, please check and retry again.");
return;
}
//Get Course codes, Credit hours and grade
String [] courseCodes = {code1,code2,code3};
String [] courseCredit = {credit1,credit2,credit3};
String [] courseGrade = {courseGrade1,courseGrade2,courseGrade3};
//Calculate total GPA points
float gpa1 = Float.parseFloat(getGpa(courseGrade1));
float gpa2 = Float.parseFloat(getGpa(courseGrade2));
float gpa3 = Float.parseFloat(getGpa(courseGrade3));
//float [] gpaArr = {gpa1,gpa2,gpa3};
float gpa1TotalPoints = gpa1 * (Float.parseFloat(credit1));
float gpa2TotalPoints = gpa2 * (Float.parseFloat(credit2));
float gpa3TotalPoints = gpa3 * (Float.parseFloat(credit3));
float [] gpaArr = {gpa1,gpa2,gpa3};
//Calculate CGPA
float cgpa1 = gpa1TotalPoints/(Float.parseFloat(credit1));
float cgpa2 = gpa2TotalPoints/(Float.parseFloat(credit2));
float cgpa3 = gpa3TotalPoints/(Float.parseFloat(credit3));
float [] cgpaArr = {cgpa1,cgpa2,cgpa3};
System.out.println("Course code \t\t\t Credit Hours \t\t\t Grade \t\t\t CGPA");
for(int x = 0; x<gpaArr.length; ++x){
System.out.println(courseCodes[x]+" \t\t\t\t "+courseCredit[x]+" \t\t\t\t "+courseGrade[x]+" \t\t\t "+cgpaArr[x]);
}
//System.out.println("Course Code"+code+" Credit Hours:"+credit+" Course Grade"+courseGrade.toUpperCase()+" ");
}
}
Program OutPut
Cite this page
Computer Science Essay Example. (2019, Sep 11). Retrieved from https://speedypaper.com/essays/group-name
Request Removal
If you are the original author of this essay and no longer wish to have it published on the SpeedyPaper website, please click below to request its removal:
- Nutrition Essay Sample: Diet Analysis with Specific Nutritional Goals
- Persuasive Book Review Essay Sample - Great Expectations by Charles Dickens
- Current Health Status of the Hispanics in the United States
- Free Essay: The Role of Social Media and the Internet in High School
- Essay Example Comprising the Math Lesson Plan
- Essay Sample on Henig and Johnson's Description on Gender Experience
- Paper Example: Appraise Staff Performance
Popular categories